In the realm of industrial automation, PLC programming languages play a pivotal role in controlling and monitoring various processes and systems. PLCs (Programmable Logic Controllers) are specialized computers designed to handle the demanding requirements of industrial environments, and the programming languages used to operate them are equally specialized and efficient.
PLC programming languages are the backbone of modern industrial automation, enabling precise control and monitoring of complex machinery, processes, and systems. These languages are specifically designed to meet the unique challenges of industrial environments, such as real-time responsiveness, reliability, and resilience. From assembly lines to power plants, PLC programming languages are essential tools for ensuring efficient and safe operations across a wide range of industries.
Ladder Logic (LD)
Ladder logic, also known as ladder diagram (LAD), is one of the most widely used PLC programming languages. It is a graphical programming language that represents program instructions in a format similar to electrical circuit diagrams. This intuitive representation makes it easier for technicians and engineers to understand and program complex control systems.
Graphical Representation
Ladder logic uses a series of rungs to represent logical operations. Each rung consists of input conditions on the left and output actions on the right. The program execution follows the path from left to right, evaluating the input conditions and executing the corresponding output actions.
Real-World Applications
Ladder logic is extensively used in various industries, including manufacturing, automotive, and process control. Its graphical nature makes it particularly suitable for programming sequential operations, such as conveyor systems, assembly line operations, and machine tool control.
Example:
| |START |STOP|
–|N/C N/O |–|MOTOR
|
In this example, the ladder rung represents the control logic for a motor. If the START input is activated and the STOP input is not activated, the MOTOR output will be energized, turning the motor on.
Structured Text (ST)
Structured Text (ST) is a textual programming language for PLCs that resembles high-level programming languages like Pascal or C. It allows for more complex program structures and control flow, making it suitable for advanced applications and algorithm implementations.
Syntax and Structure
Structured Text follows a structured programming approach, with constructs such as loops, conditional statements, and function calls. This makes it more flexible and powerful than ladder logic, enabling developers to create more complex programs.
Real-World Applications
Structured Text is often used in applications that require advanced mathematical calculations, data processing, or algorithm implementations. It is commonly found in industries like chemical processing, pharmaceutical manufacturing, and food and beverage production.
Example:
VAR
counter : INT;
END_VAR
REPEAT
counter := counter + 1;
UNTIL counter > 10
END_REPEAT;
In this example, a REPEAT loop increments the counter variable until its value exceeds 10.
Instruction List (IL)
Instruction List (IL) is a low-level textual programming language for PLCs, reminiscent of assembly language. It provides a very basic and efficient way to program PLCs, making it suitable for time-critical applications or situations where program size and execution speed are paramount.
Mnemonic Instructions
Instruction List uses mnemonic instructions to represent operations and data manipulations. These instructions are typically single-line statements, making the code compact and easy to understand for experienced programmers.
Real-World Applications
Instruction List is often used in time-critical applications where execution speed and determinism are essential, such as motion control systems, robotics, and high-speed packaging machinery.
Example:
LD 10
ADD 20
ST Result
In this example, the first instruction (LD 10) loads the value 10 into the accumulator. The second instruction (ADD 20) adds 20 to the accumulator. Finally, the third instruction (ST Result) stores the result in the Result memory location.
Sequential Function Chart (SFC)
Sequential Function Chart (SFC) is a graphical programming language for PLCs that is particularly useful for representing sequential processes and control flows. It uses a combination of steps and transitions to model the various stages and conditions of a process.
Process Representation
SFC represents a process as a series of steps, connected by transitions. Each step can contain actions or programming code, while transitions represent the conditions that must be met to proceed to the next step.
Real-World Applications
Sequential Function Chart is widely used in industries with complex sequential processes, such as batch processing, material handling, and packaging lines. It provides a clear and intuitive representation of the process flow, making it easier to understand and maintain.
Example:
INITIAL_STEP
| Conveyor_Start
|
STEP1
| Fill_Container
|
TRANSITION1
| Container_Full
|
STEP2
| Seal_Container
|
TRANSITION2
| Container_Sealed
|
FINAL_STEP
In this example, the SFC represents a process for filling and sealing containers. The process starts with the INITIAL_STEP, which initiates the conveyor. STEP1 fills the container, and TRANSITION1 checks if the container is full before proceeding to STEP2, which seals the container. TRANSITION2 verifies that the container is sealed before reaching the FINAL_STEP.
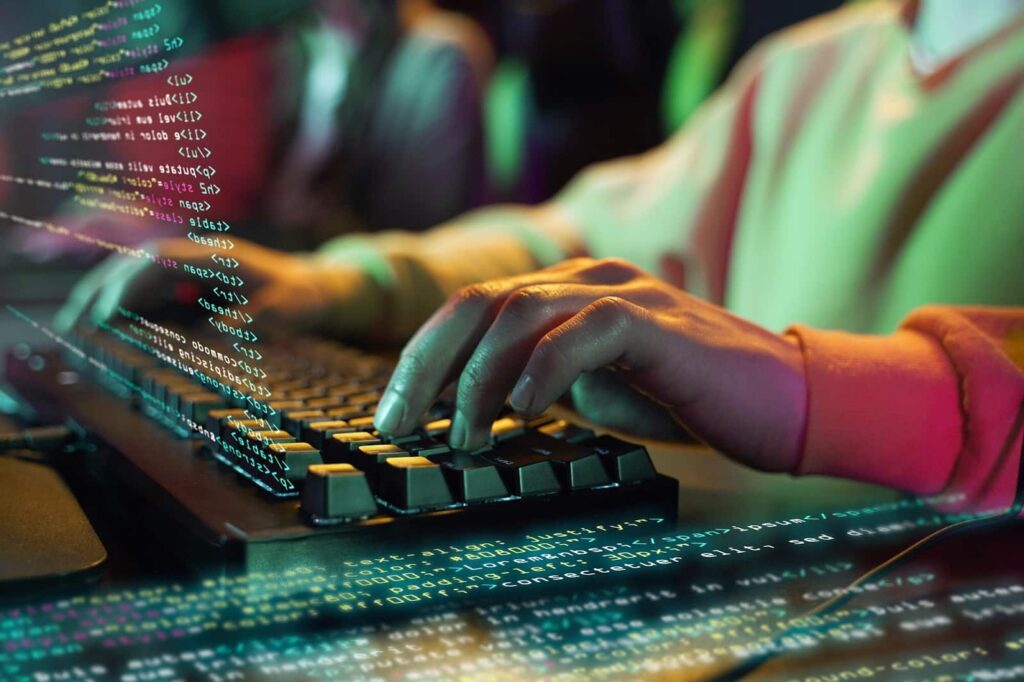
Function Block Diagram (FBD)
Function Block Diagram (FBD) is a graphical programming language for PLCs that uses a block-based approach to represent control logic. Each block represents a specific function or operation, and the connections between blocks define the data flow and control logic.
Block Representation
In FBD, blocks can represent various functions, such as logical operations (AND, OR, NOT), mathematical operations (addition, multiplication), timers, counters, and user-defined functions.
Real-World Applications
Function Block Diagram is widely used in industries that require complex control logic or data processing, such as process control, building automation, and energy management systems.
Example:
+---------------+
| |
| ADD |
| |
+-------+-------+
/ \
/ \
/ \
+----------+ +----------+
| | | |
| 10 | | 20 |
| | | |
+----------+ +----------+
In this example, the FBD represents an addition operation. The ADD block takes two inputs, 10 and 20, and performs the addition operation. The result of the addition is available as the output of the ADD block.
Human-Machine Interface (HMI)
Human-Machine Interface (HMI) programming languages are used to develop user interfaces for PLCs, allowing operators and technicians to interact with and monitor the controlled processes. HMI programming typically involves creating graphical user interfaces (GUIs) with various visual elements, such as buttons, indicators, and charts.
Visual Programming
HMI programming languages often use a visual programming approach, where developers can drag and drop various UI elements onto a canvas and configure their properties and behavior.
Real-World Applications
HMI programming languages are essential in industries that require human interaction and monitoring, such as manufacturing, oil and gas, and power generation. They provide operators with a visual representation of the process, enabling them to monitor and control various aspects of the system.
Example:
In an HMI programming environment, you might create a screen with various visual elements, such as:
- A button to start or stop a conveyor system;
- A numeric display showing the current production count;
- A chart displaying real-time temperature readings;
- An alarm indicator that lights up when a certain condition is met.
These visual elements can be configured to interact with the PLC’s control logic, allowing operators to monitor and control the process from a centralized interface.
FAQs
A PLC (Programmable Logic Controller) is a digital computer used for automating industrial processes, such as control of machinery on factory assembly lines.
A PLC (Programmable Logic Controller) is a digital computer used for automating industrial processes, such as control of machinery on factory assembly lines.
PLC programming languages are essential for programming and controlling the behavior of PLCs, which are critical components in industrial automation systems. These languages allow engineers and technicians to develop control logic, monitor processes, and ensure efficient and safe operations.
The most commonly used PLC programming languages include Ladder Logic (LD), Structured Text (ST), Instruction List (IL), Sequential Function Chart (SFC), and Function Block Diagram (FBD).
While PLC programming languages are primarily designed for industrial automation and control applications, some of these languages, such as Structured Text (ST), can be adapted for other applications that require real-time control or complex logic implementation.
The choice of PLC programming language depends on various factors, such as the complexity of the application, the required level of control and monitoring, the existing skill set of the programming team, and the specific requirements of the industry or process. It’s important to carefully evaluate these factors and consult with experts to make an informed decision.
Conclusion
PLC programming languages are the backbone of modern industrial automation, enabling precise control and monitoring of complex machinery, processes, and systems. Each language has its own strengths and applications, ranging from the intuitive graphical representations of Ladder Logic and Sequential Function Chart to the more advanced and flexible Structured Text and Function Block Diagram.
As industries continue to evolve and automation becomes increasingly crucial, the importance of PLC programming languages will only grow. By mastering these languages, engineers and technicians can unlock the full potential of industrial automation, driving efficiency, productivity, and safety across a wide range of applications.