Logic programming languages form a fascinating realm within the landscape of computer science and software development. These languages are built on the principles of mathematical logic, offering a unique approach to problem-solving and computation. By leveraging logical inference and rule-based programming, developers can create sophisticated applications that excel in domains like artificial intelligence, database systems, and expert systems. In this comprehensive guide, we will delve into the intricacies of logic programming languages, exploring their foundations, key features, popular examples, and practical applications.
Understanding Logic Programming
Logic programming traces its roots back to the early days of artificial intelligence research and mathematical logic. The concept of declarative programming, where programmers specify what needs to be done rather than how to do it, forms the core philosophy behind logic programming languages. One of the seminal languages in this domain is Prolog (Programming in Logic), which introduced the notion of defining relationships and rules to solve problems through logical inference.
Key Concepts in Logic Programming
At the heart of logic programming lies the idea of representing knowledge and solving problems using logical rules and facts. In these languages, programs consist of a set of logical statements that define relationships between entities and conditions for deriving conclusions. Unification, backtracking, and recursion are fundamental concepts that underpin the execution model of logic programming languages, enabling powerful and flexible computation strategies.
Example:
Consider a simple Prolog program that defines family relationships:
parent(john, mary).
parent(mary, ann).
ancestor(X, Y) :- parent(X, Y).
ancestor(X, Y) :- parent(X, Z), ancestor(Z, Y).
In this snippet, the ancestor predicate recursively determines if X is an ancestor of Y based on the parent relationship.
Prolog: A Pioneer in Logic Programming
Prolog stands out as one of the most widely used logic programming languages, renowned for its elegant syntax and powerful inference capabilities. Its distinctive features include pattern matching, automatic backtracking, and support for recursive programming paradigms. Prolog programs are structured around predicates and rules, allowing developers to express complex logical relationships concisely.
Practical Applications of Prolog
The versatility of Prolog has led to its adoption in various domains, ranging from natural language processing and expert systems to symbolic computation and theorem proving. Its ability to handle symbolic manipulation and rule-based reasoning makes it well-suited for tasks that involve intricate logical deductions and knowledge representation.
List of Prolog Applications:
- Expert Systems: Building intelligent systems that mimic human decision-making processes;
- Natural Language Processing: Parsing and understanding human languages for text analysis and generation;
- Symbolic Mathematics: Solving mathematical problems symbolically rather than numerically.
Application | Description |
---|---|
Expert Systems | Utilizes rule-based reasoning to provide expert-level advice or solutions. |
Natural Language Processing | Analyzes and processes human language data for various applications. |
Symbolic Mathematics | Performs mathematical computations symbolically using logical rules. |
Datalog: Logic Programming for Databases
Datalog represents a specialized form of logic programming tailored for querying and manipulating databases. It extends the principles of Prolog to facilitate data management tasks, making it an integral part of database systems and information retrieval applications. Datalog programs consist of rules that define relationships between data entities and enable efficient query processing.
Syntax and Semantics of Datalog
In Datalog, rules are expressed in a syntax similar to Prolog but with a focus on database operations like selection, projection, and join. The language emphasizes recursive queries and stratified negation to handle complex data dependencies and integrity constraints effectively. Datalog’s semantics revolve around the notion of minimal models and fixed points, ensuring soundness and completeness in query evaluation.
Example:
A basic Datalog program for querying employee information from a database:
employee(john, developer).
employee(sara, manager).
worksFor(X, Y) :- employee(X, Y).
This program defines the employee relation and a rule to determine who works for whom based on the employee data.
Answer Set Programming (ASP)
Answer Set Programming (ASP) represents a paradigm within logic programming focused on solving combinatorial search problems and knowledge representation tasks. ASP extends traditional logic programming by introducing stable model semantics and disjunctive logic programming constructs. It provides a declarative approach to defining problem domains and generating solutions through answer sets.
Applications of ASP
ASP finds applications in diverse fields such as planning, configuration, bioinformatics, and knowledge representation. Its ability to handle non-monotonic reasoning and complex problem domains makes it suitable for scenarios where traditional algorithms may struggle to provide efficient solutions. ASP models can capture intricate decision-making processes and constraints, enabling the development of intelligent systems.
List of ASP Applications:
- Automated Planning: Generating optimal plans and schedules for complex tasks;
- Bioinformatics: Analyzing biological data and genetic sequences for research purposes;
- Configuration Problems: Solving configuration puzzles and optimization challenges.
Application | Description |
---|---|
Automated Planning | Develops plans and schedules based on predefined constraints and objectives. |
Bioinformatics | Analyzes biological data to derive insights and patterns for scientific study. |
Configuration Problems | Solves configuration puzzles by determining optimal settings and arrangements. |
Constraint Logic Programming (CLP)
Constraint Logic Programming (CLP) combines logic programming with constraint satisfaction techniques to tackle problems involving complex constraints and variables. CLP languages allow developers to express both logical relationships and constraints in a unified framework, enabling efficient constraint propagation and solution finding. By integrating logical inference with constraint solving, CLP offers a versatile approach to modeling and solving constraint satisfaction problems.
Features of CLP
CLP languages provide a rich set of constraints covering domains like arithmetic, boolean logic, and finite domains. Developers can specify constraints on variables and let the underlying solver handle constraint propagation and solution derivation automatically. This declarative style of programming simplifies the task of modeling complex problems and reduces the need for explicit algorithm design.
Example:
A simple CLP program for solving a cryptarithmetic puzzle:
:- use_module(library(clpfd)).
send([S,E,N,D], [M,O,R,E], [M,O,N,E,Y]) :-
Vars = [S,E,N,D,M,O,R,Y],
Vars ins 0..9,
all_different(Vars),
S*1000 + E*100 + N*10 + D +
M*1000 + O*100 + R*10 + E
# =
M*10000 + O*1000 + N*100 + E*10 + Y,
M
# \= 0, S #\= 0.
This program uses CLP(FD) constraints to solve the classic SEND + MORE = MONEY puzzle.
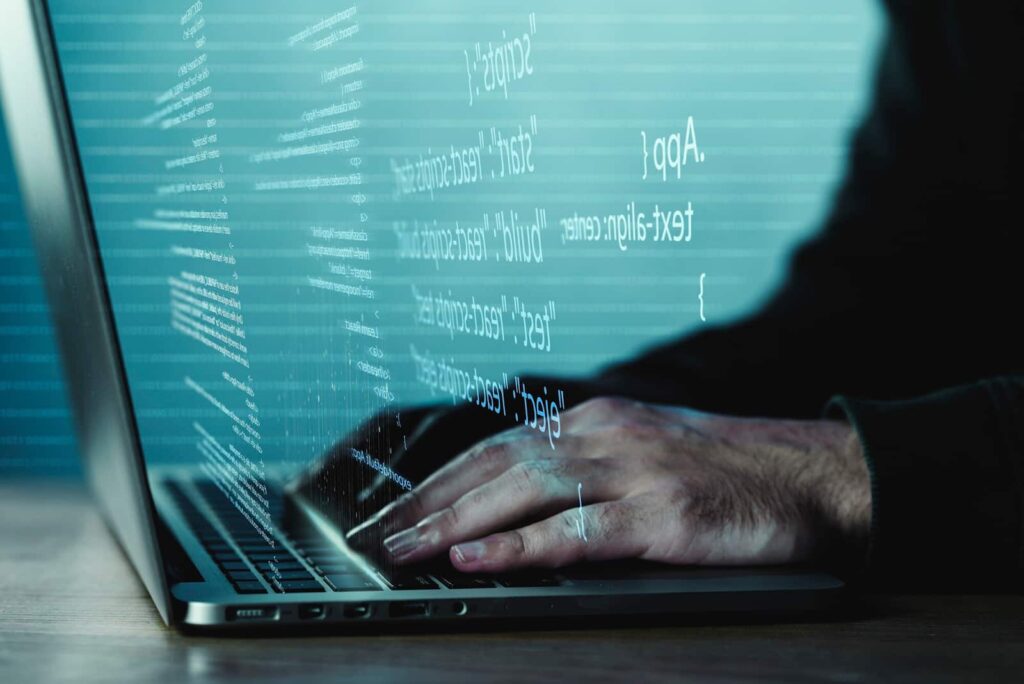
Lambda Prolog: Integrating Functional and Logic Programming
Lambda Prolog represents a fusion of functional programming principles from languages like Haskell with the logical inference capabilities of Prolog. By combining lambda calculus and logic programming constructs, Lambda Prolog enables developers to express computations in a hybrid style that leverages both functional and logic-based features. This integration enhances the expressive power of the language and facilitates the development of complex applications.
Expressiveness and Flexibility
Lambda Prolog allows for the definition of higher-order functions, pattern matching, and logical inference within a unified framework. Developers can seamlessly switch between functional and logic programming paradigms, exploiting the strengths of each approach to solve diverse problems efficiently. The language’s support for recursion, lazy evaluation, and logical quantifiers enhances its versatility in handling complex computational tasks.
List of Lambda Prolog Features:
- Higher-order Functions: Define functions that take functions as arguments or return functions;
- Pattern Matching: Match data structures against patterns for concise and expressive programming;
- Lazy Evaluation: Delay computation until the result is actually needed, improving efficiency.
Feature | Description |
---|---|
Higher-order Functions | Functions that operate on other functions as arguments or return values. |
Pattern Matching | Matching data structures against patterns for conditional processing. |
Lazy Evaluation | Deferring computation until the result is required, optimizing performance. |
FAQs
Logic programming languages offer a declarative approach to problem-solving, where programmers specify relationships and rules rather than step-by-step instructions. They excel in domains requiring complex logical reasoning and symbolic manipulation.
Prolog focuses on logical inference and rule-based programming, allowing developers to express relationships and constraints declaratively. In contrast, imperative languages emphasize sequential execution and explicit control flow.
Datalog is commonly used in database systems for querying and data manipulation tasks. It also finds applications in information retrieval, network analysis, and semantic web technologies.
Yes, ASP is well-suited for solving complex combinatorial search problems due to its ability to represent constraints and generate solutions through stable model semantics. It is used in various domains requiring intelligent decision-making.
Constraint Logic Programming integrates logical inference with constraint solving techniques, allowing developers to model complex constraints and variables in a unified framework. This approach simplifies the process of solving constraint satisfaction problems efficiently.
Conclusion
Logic programming languages represent a diverse and powerful set of tools for addressing complex computational challenges. From the foundational principles of Prolog to specialized variants like Datalog, ASP, CLP, and Lambda Prolog, these languages offer unique approaches to problem-solving that cater to a wide range of applications. By embracing logical inference, rule-based reasoning, and constraint satisfaction techniques, developers can leverage the expressive nature of logic programming to build intelligent systems, solve intricate puzzles, and model complex domains effectively. As the field of computer science continues to evolve, logic programming languages remain at the forefront of innovation, driving advancements in artificial intelligence, database systems, and beyond.